Inlining in Go
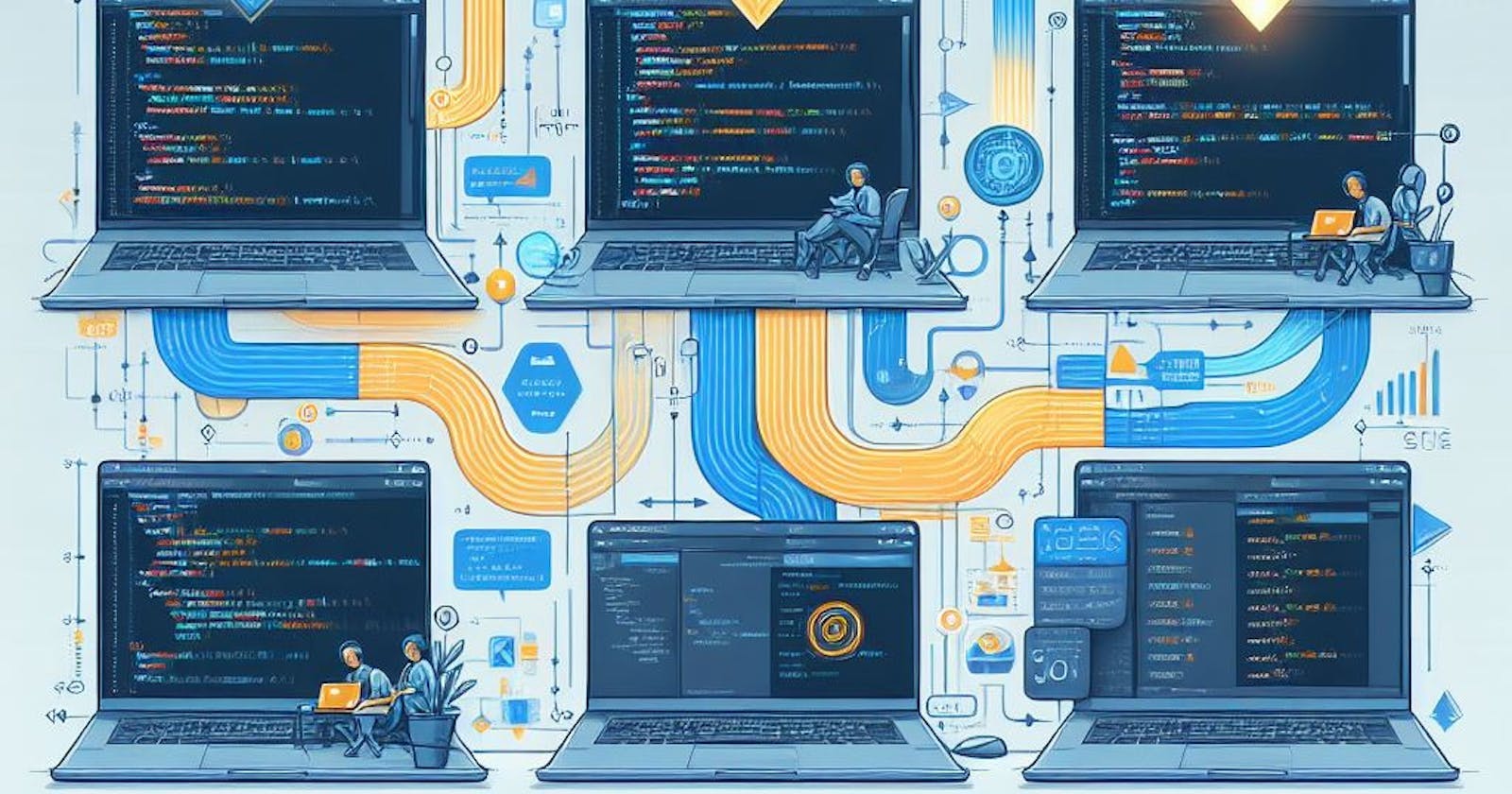
The process of grouping smaller functions into their respective callers in Go Compiler is known as inlining. This optimization was often done by hand in the early days of computing. Inlining is now one of the essential optimizations that are executed automatically throughout the compilation process.
Function Identification: The compiler scans your code, identifying functions suitable for inlining. It prioritizes smaller, frequently called functions with simple bodies.
Eliminating Overhead: Instead of generating a separate function call, the compiler replaces the call with the function's code, removing the overhead of:
Code Integration: The inlined function's code is seamlessly integrated into the caller's code, creating a single, streamlined block.
flowchart TB
X[1. Start compile]
X-->SC
F[Executable bin]
subgraph SC[Source Code main.go]
subgraph A[Function main]
D[function main Body]
end
subgraph B["function a()"]
C["function a() body"]
end
A--2. Call(1) -->B
C--3. Append (inlining)-->D
end
SC--4. build --> F
func add(x, y int) int {
return x + y
}
func main() {
result := add(5, 3) // Function call
// ...
}
After inlining:
func main() {
result := 5 + 3 // Inlined code
// ...
}
Function inlining is a potent optimization tool in Go, but its judicious application is crucial. Understanding its mechanics and trade-offs empowers you to write efficient and maintainable Go code. By carefully considering function design, profiling performance, and leveraging compiler hints when necessary, you can effectively harness the power of inlining for optimal code execution.